Exploration Robot
Orientation
Motivation
The application areas of simulation in modern industrial systems range from Digital Twin to autonomous transport systems, production machinery, additive manufacturing and many more. Simulation offers a cost-effective, safe and fast analysis tool to minimize cost, downtime and transportation time. This is done by providing a painless way of running different algorithms, machinery setup or production sequences without the need to change the real world setup. Since simulation is becoming more and more important and widespread, it is indispensable gather knowledge in this area. The widespread use also has the advantage that ready-made software already exist for many operating systems. The aim of this online exericise is to discover a virtual maze by simulating a robot.
Requirements
- Knowledge of a programming language is necessary (C/C++, Python, ...).
- Knowledge of the Robot Operating System (ROS) is necessary (ROS tutorial).
- Knowledge of object-oriented programming is recommended, but not necessary.
Goals
After completing the exercise, you will be able to…
- … work with the physics simulation engine GAZEBO in combination with ROS.
- … develop an understanding of data-structure in ROS.
- … explore a simulated maze using a mobile robot.
Recommended Reading
- The basics in the next section
- The ROS wiki page: wiki.ros.org/
Guide
The remaining part of this page gives a brief overview on the simulation, installation and exercise walk-through.
Before the online exericse can be started, a manual is required, which can be downloaded HERE.
The document Manual.pdf contains detailed descriptions of the individual steps and, hence, should be used during the whole exercise execution.
There are also several references to the manual on this website.
The exercise takes between 60 and 90 minutes.
The concrete duration depends on the individual learning process.
The following activities are expected of you:
- In the module Basics
- read up on and develop an understanding of the necessary theory.
- In the module Exercise
- read the general conditions of the exercise.
- In the module Application
- you will be given a task.
- In the module Considerations
- you get a short summary of the results.
Basics
Simulation Description
This chapter introduces the simulation environment as well as the simulated mobile robot Turtlebot3 Burger.
Mobile Robot - Turtlebot3 Burger
The TurtleBot3 Burger is a cost-effective, personalized robot kit driven using open source software developed by Robotis Bioloid. It is equipped with necessary sensors to build a map of the environment and operate in it. The following figure visualizes the components of the Turtlebot3 Burger.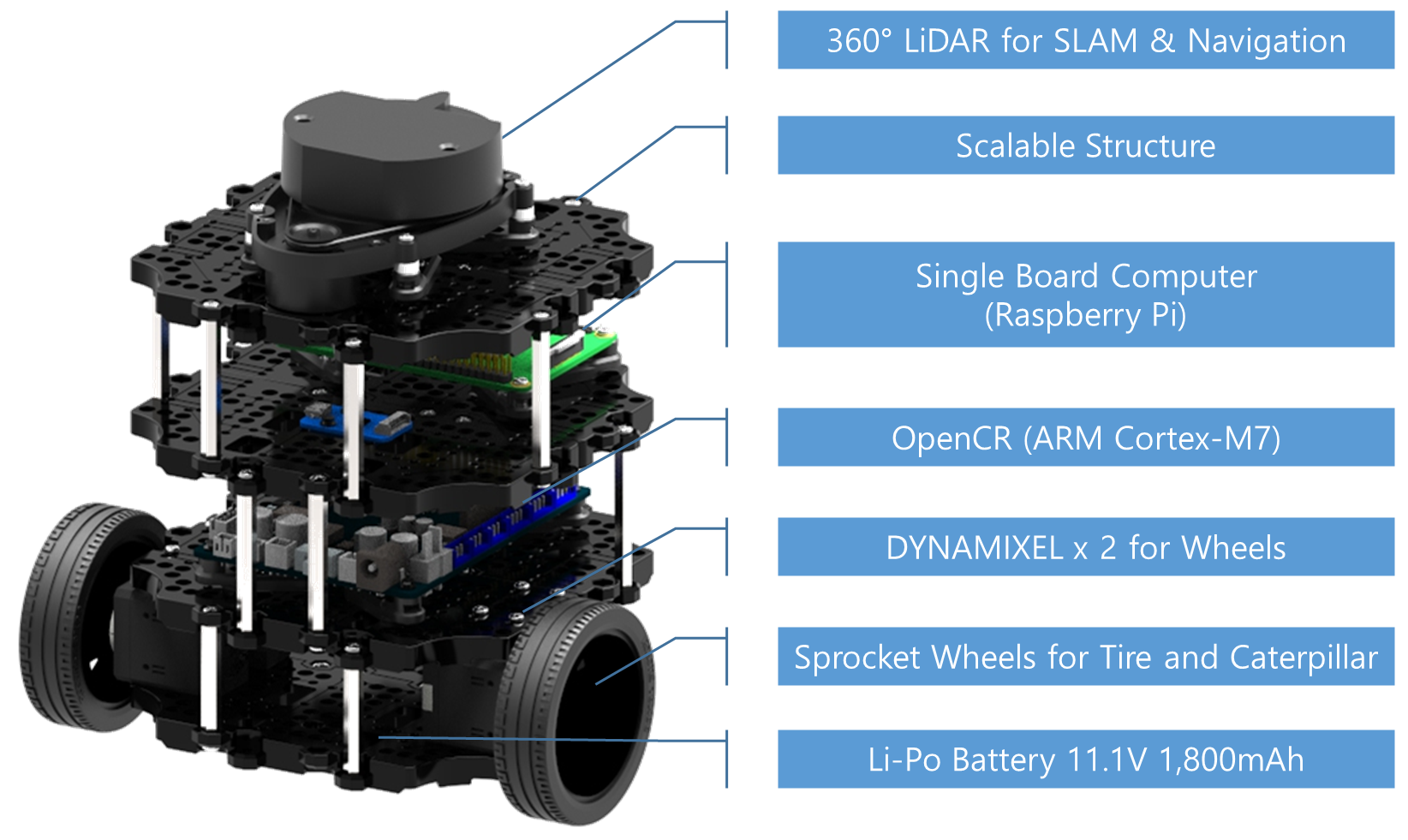
The 360°LIDAR is utilized during this exercise to build a map of the environment using gmapping as well as to localize itself at the same time using Advanced Monte Carlo Localization (AMCL). This is known as the Simultaneous Localization and Mapping (SLAM) problem.
Simulation Environment - GAZEBO
Robot simulation is an indispensable tool in the toolbox of every robotic engineer. A well designed simulator allows you to quickly test algorithms, design robots, perform regression tests and train AI systems using realistic scenarios. Gazebo provides the ability to precisely and efficiently simulate populations of robots in complex indoor and outdoor environments. A robust physics engine, high-quality graphics and comfortable programmatic and graphical interfaces are available.This exercises utilizes GAZEBO as a physics simulation engine to simulate the above mentioned actuators and sensors of the mobile robot as well as a automatically generated maze. The next figure visualizes a running simulation of said maze and mobile robot. Note that when you start the explorer node as described below (see 3. Setup) this Graphical User Interface of GAZEBO will not be opened due to performance optimisation, instead RVIZ will be opened to visualize the sensor readings and the environment.
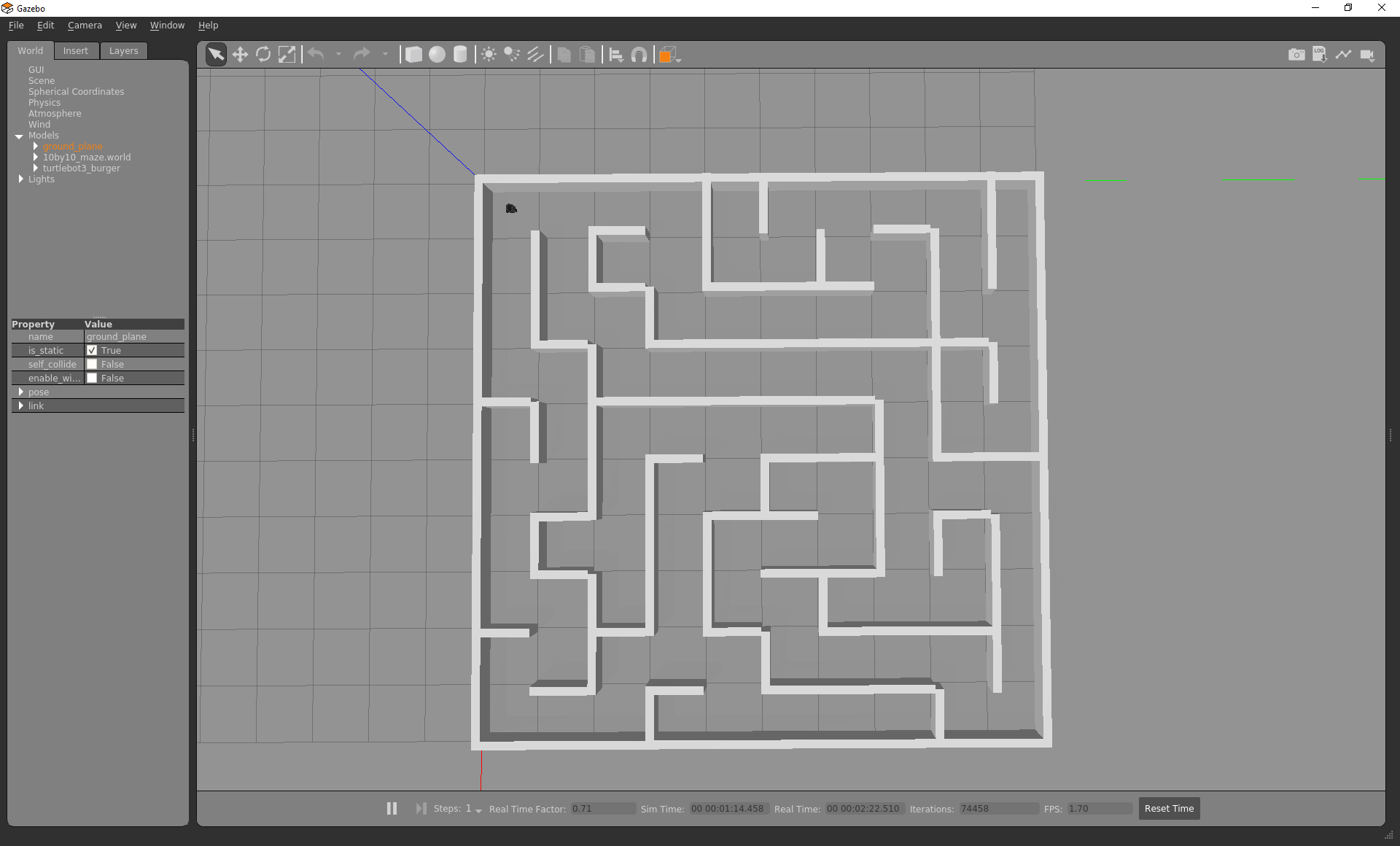
Explorer Algorithms
This chapter introduces pseudo codes of the explorer algorithms Rapidly Random Search Tree Exploration (RRT) and Wall Follow Exploration (WF).
These algorithms can also be adapted or substituted when working on the application of this online exercise.
Independently which algorithm is used, keep in mind, that the LIDAR systems used on the Turtlebot3 Burger has a maximum range of 3.5m
Rapidly Random Search Tree Exploration
RRT is a search algorithm that randomly searches high-dimensional search spaces for possible paths. In robotics, the algorithm and its variations are often used for motion planning or exploration tasks. The following pseudo code gives an overview of the steps needed to implement an RRT for exploration.procedure RRT {
for < itr <= MAXitr > {
N = <n; get_position()i> #N visited nodes as vector
i ~ U(0,N) #Take random sample from LIDAR msg M
P = gen_pose(M[i]) #Generate a pose using the sample and M
p = transform_pose(P) #Transform P to map coordinate frame
if < compare_nodes(p; N) > {
sendGoal(p)
while < curr_time - start_time <= timeout > {
wait_for_feedback()
}
}
}
}
Wall Follow Exploration
Exploration strategies differ in the extent to which the development map is used to control the explorative movements. Wall trackers solely rely only on the measurements of distance sensors, which measure the distances to the walls to explore an environment. The following pseudo code gives an overview of the steps needed to impement a wall follower for exploration.procedure FOLLOW_WALL {
find_nearest_wall(M) #Find the nearest wall using the LIDAR msg M
drive_to_nearest_wall(M) #Geometrically calculate the desired distance to the wall and publish it.
while < itr <= MAXitr > {
calc_next_pose() #Here is the actual wall following executed
itr++
}
}
Exercise
Download Required Software
Docker
This software is required under Windows and MacOS. After downloading the software (HERE, it can be installed as usual.X11-Server
This software is only required under Windows and can be downloaded HERE and installed afterwards.ROS Package
This is an additional software (necessary for all operating systems), which is required for the successful build of the Docker container and can be downloaded HERE. The content of the packed file (ROS_Gazebo.zip) is explained in chapters 1.3, 1.4 as well as 1.5 in the manual (Manual.pdf). Following steps are required for the installation:- unpack the folder
- double click on build_docker_container.bat
Running Software
Docker
The software is easily started over the program Docker Desktop, which will be shown as a notification.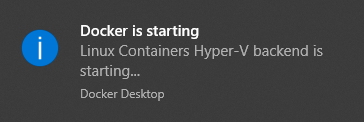
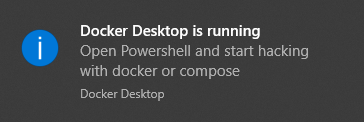
X11-Server
As described earlier, this software is only necessary under windows. Therefore, the program XLaunch is started. Then, a window with settings pops up, while the default settings can be confirmed by clicking on Next. All presettings, except those in the window with title Extra Settings, can be confirmed. The required extra settings must be used as illustrated in following configuration.ROS Package
The final step, before starting with programming, is to start the Docker container. This is done by executing following steps:- double click on run_docker.bat
- At the first start, Docker requires permissions to mount the folder catkin_ws in the Docker container. This is required in order to avoid the loss of modifications done, when closing the container.
- Start programming!
Exploring Maze
After installing and starting the required software, the simulation of the Turtlebot3 Burger can be started.
This chapter describes, how a pre-assembled program code of the ROS package is started for the simulation exercise.The first step is to generate a Docker container. Then, the simulaiton can be started by the command start-maze.sh. After the row "Finished maze generation, Press enter to start gazebo" appears in the terminal, the whole simulation is started by confirming with the return key. This should lead to the generation of several windows, what is shown in the following image.
The sample code only works until the catkin_ws workspace is built for the first time, because then the binary files are overwritten. Implement and test your explorer algorithm! A detailed explanation on how to test an algorithm is found in the file example.hpp, which is located in ROS_Gazebo\catkin_ws\src\fhtw_maze_explorer\include\fhtw_maze_explorer.
Application
The example solution in the previous chapters demonstrated how the simulation is started correctly.
The final result of the task, i.e. the complete maze, can thereby explored completely.
Since the algorithm is not accessible, a self-written algorithm shall be used in order to explore the maze.
The type of explorer algorithm is arbitrary.
A runtime comparison between different explorer algorithm can be done additionally.The following description guides through the required steps in order to generate and test new implemented algorithms.
- New algorithms must be implemented inside the file example.hpp, which is located in the /include/fhtw_explorer/ directiory. This file overwrites the Base Class Function, what allows the usage of new algorithms. Furhter details are found in the file as commands.
- For testing/starting new algorithms from the file example.hpp, following commands must be executed:
- cd /root/catkin_ws
Command to change to the catkin directory - catkin_make
Command to generate the working range and the fhtw_maze_explorer package - start-maze.sh [-Options]
Command to start the maze generator as well as GAZEBO, RVIZ and the ROS node with following options:
-s: maze size 10x10
-m: maze size 25x25
-l: maze size 50x50
-d: debug of the maze_explorer
- cd /root/catkin_ws
Considerations
After completing the exercise, you are able to…
- … work with the physics simulation engine GAZEBO in combination with ROS.
- … develop an understanding of data-structure in ROS.
- … explore a simulated maze using a mobile robot.
Self-Evaluation
Below you find questions, which you can solve after succeeding the online exercise. The solutions are shown by clicking on the questions.
What is the difference between actions and services in ROS?
An action is an asynchronous activity, which allows to do other things until the result of the request is obtained. In contrast, services represent synchronous activities. Hence, one has to wait for the result before it is possible to do other things. Deshalb wird auch bei Services von blocking und bei Actions von non-blocking Aktivitäten gesprochen. Therefore, services are called blocking and actions non-blocking activities.
How are LaserScan messages structured?
LaserScan ist ein spezielles Format, das für Roboter mit Laserscannern eine einfache Methode zur Informationsaufbereitung bietet.
Es handelt sich um einen Message-Typen, der folgendermaßen strukturiert ist:
LaserScan is a special format, which provides a simple information processing method for robots with laser scans.
LaserScan is of type message, which is structured as follows:
- Header header (timestamp of the initially emitted laser beam)
- float32 angle_min (start angle of the scan)
- float32 angle_max (end angle of the scan)
- float32 angle_increment (time between measurements)
- float32 scan_time (time between scans)
- float32 range_min (minimum range value)
- float32 range_max (maximum range value)
- float32[] ranges (range data)
- float32[] intensities (intensity data)
How does the debug of ROS nodes work?
Since ROS nodes run as processes with a PID in the operating system, the debug can be executed with standard tools. E.g. the UNIX program gdb can be used.
How can rosnode info help when debugging ROS nodes?
By executing the command rosnode_info
Take-Home-Messages
- By using Docker containers, application packages can be easiliy transported without any installation as well as provided on different systems.
- Simulations help with the implementation of software for real hardware, what saves costs and development time.
- There are different algorithms, which can be used for the automated environment exploration. These differ in complexity as well as required runtime for the whole mapping.
- LiDAR sensors on mobile robots are optimally suited in order to detect surrounding walls and generate maps thereby.
- SLAM (Simultaneous Localization And Mapping) describes the simultaneous environmental mapping as well as localization.
Links and Literaure
- Download Manual.pdf
- Robot Operating System (ROS)
- ROS Tutorial
- ROS Wiki
- Robotis Bioloid
- gmapping
- Advanced Monte Carlo Localization
- Simultaneous Localization and Mapping
- Download Docker
- Download X11-Server
- Download ROS Package
Further Topics
Maze Solving Algorithms
Descriptions of different explorer algorithmsGAZEBO - weiterführende Informationen
Description how development environments for simulations (also in 3D) are generatedCatkin - weiterführende Informationen
Catkin explanation and tutorial